Creating nested elements dynamically
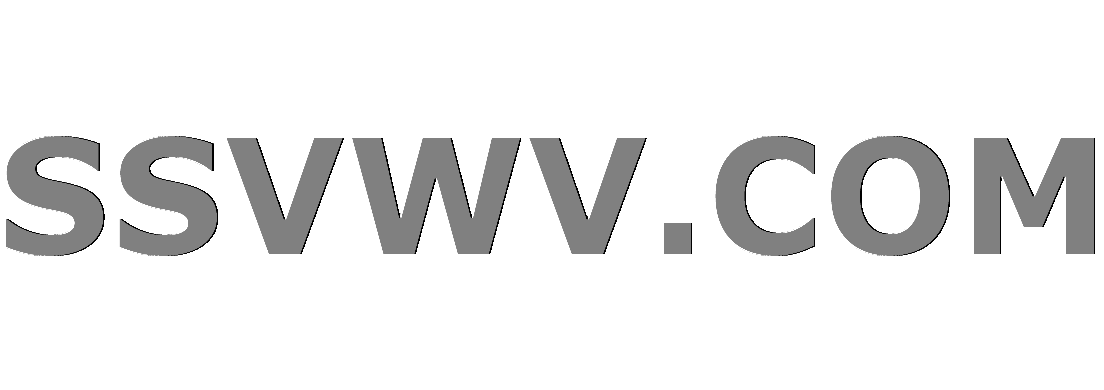
Multi tool use
I have this array of strings var arr = ["ul", "li", "strong", "em", "u"]
.
How can I make these into DOM Elements one inside another from left to right, first element as the root element. Without using ID because of some reason.
And maybe by using loop for it to be flexible to any number of elements.
var new_element = document.createElement(arr[0]);
I'm expecting something like this:
<ul>
<li><strong><em><u>Text Here</u></em></strong></li>
</ul>
Thank you.
javascript html dom
add a comment |
I have this array of strings var arr = ["ul", "li", "strong", "em", "u"]
.
How can I make these into DOM Elements one inside another from left to right, first element as the root element. Without using ID because of some reason.
And maybe by using loop for it to be flexible to any number of elements.
var new_element = document.createElement(arr[0]);
I'm expecting something like this:
<ul>
<li><strong><em><u>Text Here</u></em></strong></li>
</ul>
Thank you.
javascript html dom
1
You want to know aboutNode.appendChild
.
– moonwave99
2 days ago
add a comment |
I have this array of strings var arr = ["ul", "li", "strong", "em", "u"]
.
How can I make these into DOM Elements one inside another from left to right, first element as the root element. Without using ID because of some reason.
And maybe by using loop for it to be flexible to any number of elements.
var new_element = document.createElement(arr[0]);
I'm expecting something like this:
<ul>
<li><strong><em><u>Text Here</u></em></strong></li>
</ul>
Thank you.
javascript html dom
I have this array of strings var arr = ["ul", "li", "strong", "em", "u"]
.
How can I make these into DOM Elements one inside another from left to right, first element as the root element. Without using ID because of some reason.
And maybe by using loop for it to be flexible to any number of elements.
var new_element = document.createElement(arr[0]);
I'm expecting something like this:
<ul>
<li><strong><em><u>Text Here</u></em></strong></li>
</ul>
Thank you.
javascript html dom
javascript html dom
edited 2 days ago
Community♦
11
11
asked 2 days ago
MuyieMuyie
728
728
1
You want to know aboutNode.appendChild
.
– moonwave99
2 days ago
add a comment |
1
You want to know aboutNode.appendChild
.
– moonwave99
2 days ago
1
1
You want to know about
Node.appendChild
.– moonwave99
2 days ago
You want to know about
Node.appendChild
.– moonwave99
2 days ago
add a comment |
3 Answers
3
active
oldest
votes
You can do this with reduceRight()
which avoids needing to query the result to get the deepest value because it starts with the deepest element and works out. The return value will be the topmost element:
var arr = ["ul", "li", "strong", "em", "u"]
let el = arr.reduceRight((el, n) => {
let d = document.createElement(n)
d.appendChild(el)
return d
}, document.createTextNode("Text Here"))
document.getElementById('container').appendChild(el)
<div id = "container"></div>
It also fails gracefully with an empty array:
var arr =
let el = arr.reduceRight((el, n) => {
let d = document.createElement(n)
d.appendChild(el)
return d
}, document.createTextNode("Text Here"))
document.getElementById('container').appendChild(el)
<div id = "container"></div>
1
Great! Works as expected. I'll be analyzing how this works and read about the reduce and reduceRight function. Thank you
– Muyie
2 days ago
add a comment |
You can use Array.prototype.reduce
and Node.prototype.appendChild
.
https://jsbin.com/hizetekato/edit?html,js,output
var arr = ["ul", "li", "strong", "em", "u"];
function createChildren(mount, arr) {
return arr.reduce((parent, elementName, i) => {
const element = document.createElement(elementName);
parent.appendChild(element);
return element;
}, mount);
}
const deepest = createChildren(document.querySelector('#root'), arr);
deepest.innerText = 'WebDevNSK'
<div id="root"></div>
Seems like this is what I am looking for. I'll try to analyze it. And read about .reduce() Thank you btw.
– Muyie
2 days ago
What about=> parent.appendChild(document.createElement(elementName));
?Node.appendChild
returns the appended child. However, readability is probably more important that concision.
– Solomon Ucko
2 days ago
add a comment |
createDocumentFragment()
and run the array through a loop. On each iteration createElement()
and appendChild()
const frag = document.createDocumentFragment();
const useless = ["ul", "li", "strong", "em", "u"];
let previous;
for (let u = 0; u < useless.length; u++) {
const current = document.createElement(useless[u]);
if (u === 0) {
frag.appendChild(current);
} else {
previous.appendChild(current);
}
previous = current;
}
document.body.appendChild(frag);
ul {
outline: 5px dashed green;
padding: 15px;
}
li {
outline: 5px solid blue;
padding: 12px;
}
strong {
outline: 5px dashed red;
padding: 9px
}
em {
outline: 5px dashed grey;
padding: 6px
}
u {
outline: 5px solid black;
padding: 3px;
}
u::before {
content: 'THIS TEXT SHOULD BE UNDERLINED'
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55311908%2fcreating-nested-elements-dynamically%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can do this with reduceRight()
which avoids needing to query the result to get the deepest value because it starts with the deepest element and works out. The return value will be the topmost element:
var arr = ["ul", "li", "strong", "em", "u"]
let el = arr.reduceRight((el, n) => {
let d = document.createElement(n)
d.appendChild(el)
return d
}, document.createTextNode("Text Here"))
document.getElementById('container').appendChild(el)
<div id = "container"></div>
It also fails gracefully with an empty array:
var arr =
let el = arr.reduceRight((el, n) => {
let d = document.createElement(n)
d.appendChild(el)
return d
}, document.createTextNode("Text Here"))
document.getElementById('container').appendChild(el)
<div id = "container"></div>
1
Great! Works as expected. I'll be analyzing how this works and read about the reduce and reduceRight function. Thank you
– Muyie
2 days ago
add a comment |
You can do this with reduceRight()
which avoids needing to query the result to get the deepest value because it starts with the deepest element and works out. The return value will be the topmost element:
var arr = ["ul", "li", "strong", "em", "u"]
let el = arr.reduceRight((el, n) => {
let d = document.createElement(n)
d.appendChild(el)
return d
}, document.createTextNode("Text Here"))
document.getElementById('container').appendChild(el)
<div id = "container"></div>
It also fails gracefully with an empty array:
var arr =
let el = arr.reduceRight((el, n) => {
let d = document.createElement(n)
d.appendChild(el)
return d
}, document.createTextNode("Text Here"))
document.getElementById('container').appendChild(el)
<div id = "container"></div>
1
Great! Works as expected. I'll be analyzing how this works and read about the reduce and reduceRight function. Thank you
– Muyie
2 days ago
add a comment |
You can do this with reduceRight()
which avoids needing to query the result to get the deepest value because it starts with the deepest element and works out. The return value will be the topmost element:
var arr = ["ul", "li", "strong", "em", "u"]
let el = arr.reduceRight((el, n) => {
let d = document.createElement(n)
d.appendChild(el)
return d
}, document.createTextNode("Text Here"))
document.getElementById('container').appendChild(el)
<div id = "container"></div>
It also fails gracefully with an empty array:
var arr =
let el = arr.reduceRight((el, n) => {
let d = document.createElement(n)
d.appendChild(el)
return d
}, document.createTextNode("Text Here"))
document.getElementById('container').appendChild(el)
<div id = "container"></div>
You can do this with reduceRight()
which avoids needing to query the result to get the deepest value because it starts with the deepest element and works out. The return value will be the topmost element:
var arr = ["ul", "li", "strong", "em", "u"]
let el = arr.reduceRight((el, n) => {
let d = document.createElement(n)
d.appendChild(el)
return d
}, document.createTextNode("Text Here"))
document.getElementById('container').appendChild(el)
<div id = "container"></div>
It also fails gracefully with an empty array:
var arr =
let el = arr.reduceRight((el, n) => {
let d = document.createElement(n)
d.appendChild(el)
return d
}, document.createTextNode("Text Here"))
document.getElementById('container').appendChild(el)
<div id = "container"></div>
var arr = ["ul", "li", "strong", "em", "u"]
let el = arr.reduceRight((el, n) => {
let d = document.createElement(n)
d.appendChild(el)
return d
}, document.createTextNode("Text Here"))
document.getElementById('container').appendChild(el)
<div id = "container"></div>
var arr = ["ul", "li", "strong", "em", "u"]
let el = arr.reduceRight((el, n) => {
let d = document.createElement(n)
d.appendChild(el)
return d
}, document.createTextNode("Text Here"))
document.getElementById('container').appendChild(el)
<div id = "container"></div>
var arr =
let el = arr.reduceRight((el, n) => {
let d = document.createElement(n)
d.appendChild(el)
return d
}, document.createTextNode("Text Here"))
document.getElementById('container').appendChild(el)
<div id = "container"></div>
var arr =
let el = arr.reduceRight((el, n) => {
let d = document.createElement(n)
d.appendChild(el)
return d
}, document.createTextNode("Text Here"))
document.getElementById('container').appendChild(el)
<div id = "container"></div>
answered 2 days ago


Mark MeyerMark Meyer
39.6k33162
39.6k33162
1
Great! Works as expected. I'll be analyzing how this works and read about the reduce and reduceRight function. Thank you
– Muyie
2 days ago
add a comment |
1
Great! Works as expected. I'll be analyzing how this works and read about the reduce and reduceRight function. Thank you
– Muyie
2 days ago
1
1
Great! Works as expected. I'll be analyzing how this works and read about the reduce and reduceRight function. Thank you
– Muyie
2 days ago
Great! Works as expected. I'll be analyzing how this works and read about the reduce and reduceRight function. Thank you
– Muyie
2 days ago
add a comment |
You can use Array.prototype.reduce
and Node.prototype.appendChild
.
https://jsbin.com/hizetekato/edit?html,js,output
var arr = ["ul", "li", "strong", "em", "u"];
function createChildren(mount, arr) {
return arr.reduce((parent, elementName, i) => {
const element = document.createElement(elementName);
parent.appendChild(element);
return element;
}, mount);
}
const deepest = createChildren(document.querySelector('#root'), arr);
deepest.innerText = 'WebDevNSK'
<div id="root"></div>
Seems like this is what I am looking for. I'll try to analyze it. And read about .reduce() Thank you btw.
– Muyie
2 days ago
What about=> parent.appendChild(document.createElement(elementName));
?Node.appendChild
returns the appended child. However, readability is probably more important that concision.
– Solomon Ucko
2 days ago
add a comment |
You can use Array.prototype.reduce
and Node.prototype.appendChild
.
https://jsbin.com/hizetekato/edit?html,js,output
var arr = ["ul", "li", "strong", "em", "u"];
function createChildren(mount, arr) {
return arr.reduce((parent, elementName, i) => {
const element = document.createElement(elementName);
parent.appendChild(element);
return element;
}, mount);
}
const deepest = createChildren(document.querySelector('#root'), arr);
deepest.innerText = 'WebDevNSK'
<div id="root"></div>
Seems like this is what I am looking for. I'll try to analyze it. And read about .reduce() Thank you btw.
– Muyie
2 days ago
What about=> parent.appendChild(document.createElement(elementName));
?Node.appendChild
returns the appended child. However, readability is probably more important that concision.
– Solomon Ucko
2 days ago
add a comment |
You can use Array.prototype.reduce
and Node.prototype.appendChild
.
https://jsbin.com/hizetekato/edit?html,js,output
var arr = ["ul", "li", "strong", "em", "u"];
function createChildren(mount, arr) {
return arr.reduce((parent, elementName, i) => {
const element = document.createElement(elementName);
parent.appendChild(element);
return element;
}, mount);
}
const deepest = createChildren(document.querySelector('#root'), arr);
deepest.innerText = 'WebDevNSK'
<div id="root"></div>
You can use Array.prototype.reduce
and Node.prototype.appendChild
.
https://jsbin.com/hizetekato/edit?html,js,output
var arr = ["ul", "li", "strong", "em", "u"];
function createChildren(mount, arr) {
return arr.reduce((parent, elementName, i) => {
const element = document.createElement(elementName);
parent.appendChild(element);
return element;
}, mount);
}
const deepest = createChildren(document.querySelector('#root'), arr);
deepest.innerText = 'WebDevNSK'
<div id="root"></div>
var arr = ["ul", "li", "strong", "em", "u"];
function createChildren(mount, arr) {
return arr.reduce((parent, elementName, i) => {
const element = document.createElement(elementName);
parent.appendChild(element);
return element;
}, mount);
}
const deepest = createChildren(document.querySelector('#root'), arr);
deepest.innerText = 'WebDevNSK'
<div id="root"></div>
var arr = ["ul", "li", "strong", "em", "u"];
function createChildren(mount, arr) {
return arr.reduce((parent, elementName, i) => {
const element = document.createElement(elementName);
parent.appendChild(element);
return element;
}, mount);
}
const deepest = createChildren(document.querySelector('#root'), arr);
deepest.innerText = 'WebDevNSK'
<div id="root"></div>
edited 2 days ago
Jack Bashford
13.1k31847
13.1k31847
answered 2 days ago
dangreendangreen
1498
1498
Seems like this is what I am looking for. I'll try to analyze it. And read about .reduce() Thank you btw.
– Muyie
2 days ago
What about=> parent.appendChild(document.createElement(elementName));
?Node.appendChild
returns the appended child. However, readability is probably more important that concision.
– Solomon Ucko
2 days ago
add a comment |
Seems like this is what I am looking for. I'll try to analyze it. And read about .reduce() Thank you btw.
– Muyie
2 days ago
What about=> parent.appendChild(document.createElement(elementName));
?Node.appendChild
returns the appended child. However, readability is probably more important that concision.
– Solomon Ucko
2 days ago
Seems like this is what I am looking for. I'll try to analyze it. And read about .reduce() Thank you btw.
– Muyie
2 days ago
Seems like this is what I am looking for. I'll try to analyze it. And read about .reduce() Thank you btw.
– Muyie
2 days ago
What about
=> parent.appendChild(document.createElement(elementName));
? Node.appendChild
returns the appended child. However, readability is probably more important that concision.– Solomon Ucko
2 days ago
What about
=> parent.appendChild(document.createElement(elementName));
? Node.appendChild
returns the appended child. However, readability is probably more important that concision.– Solomon Ucko
2 days ago
add a comment |
createDocumentFragment()
and run the array through a loop. On each iteration createElement()
and appendChild()
const frag = document.createDocumentFragment();
const useless = ["ul", "li", "strong", "em", "u"];
let previous;
for (let u = 0; u < useless.length; u++) {
const current = document.createElement(useless[u]);
if (u === 0) {
frag.appendChild(current);
} else {
previous.appendChild(current);
}
previous = current;
}
document.body.appendChild(frag);
ul {
outline: 5px dashed green;
padding: 15px;
}
li {
outline: 5px solid blue;
padding: 12px;
}
strong {
outline: 5px dashed red;
padding: 9px
}
em {
outline: 5px dashed grey;
padding: 6px
}
u {
outline: 5px solid black;
padding: 3px;
}
u::before {
content: 'THIS TEXT SHOULD BE UNDERLINED'
}
add a comment |
createDocumentFragment()
and run the array through a loop. On each iteration createElement()
and appendChild()
const frag = document.createDocumentFragment();
const useless = ["ul", "li", "strong", "em", "u"];
let previous;
for (let u = 0; u < useless.length; u++) {
const current = document.createElement(useless[u]);
if (u === 0) {
frag.appendChild(current);
} else {
previous.appendChild(current);
}
previous = current;
}
document.body.appendChild(frag);
ul {
outline: 5px dashed green;
padding: 15px;
}
li {
outline: 5px solid blue;
padding: 12px;
}
strong {
outline: 5px dashed red;
padding: 9px
}
em {
outline: 5px dashed grey;
padding: 6px
}
u {
outline: 5px solid black;
padding: 3px;
}
u::before {
content: 'THIS TEXT SHOULD BE UNDERLINED'
}
add a comment |
createDocumentFragment()
and run the array through a loop. On each iteration createElement()
and appendChild()
const frag = document.createDocumentFragment();
const useless = ["ul", "li", "strong", "em", "u"];
let previous;
for (let u = 0; u < useless.length; u++) {
const current = document.createElement(useless[u]);
if (u === 0) {
frag.appendChild(current);
} else {
previous.appendChild(current);
}
previous = current;
}
document.body.appendChild(frag);
ul {
outline: 5px dashed green;
padding: 15px;
}
li {
outline: 5px solid blue;
padding: 12px;
}
strong {
outline: 5px dashed red;
padding: 9px
}
em {
outline: 5px dashed grey;
padding: 6px
}
u {
outline: 5px solid black;
padding: 3px;
}
u::before {
content: 'THIS TEXT SHOULD BE UNDERLINED'
}
createDocumentFragment()
and run the array through a loop. On each iteration createElement()
and appendChild()
const frag = document.createDocumentFragment();
const useless = ["ul", "li", "strong", "em", "u"];
let previous;
for (let u = 0; u < useless.length; u++) {
const current = document.createElement(useless[u]);
if (u === 0) {
frag.appendChild(current);
} else {
previous.appendChild(current);
}
previous = current;
}
document.body.appendChild(frag);
ul {
outline: 5px dashed green;
padding: 15px;
}
li {
outline: 5px solid blue;
padding: 12px;
}
strong {
outline: 5px dashed red;
padding: 9px
}
em {
outline: 5px dashed grey;
padding: 6px
}
u {
outline: 5px solid black;
padding: 3px;
}
u::before {
content: 'THIS TEXT SHOULD BE UNDERLINED'
}
const frag = document.createDocumentFragment();
const useless = ["ul", "li", "strong", "em", "u"];
let previous;
for (let u = 0; u < useless.length; u++) {
const current = document.createElement(useless[u]);
if (u === 0) {
frag.appendChild(current);
} else {
previous.appendChild(current);
}
previous = current;
}
document.body.appendChild(frag);
ul {
outline: 5px dashed green;
padding: 15px;
}
li {
outline: 5px solid blue;
padding: 12px;
}
strong {
outline: 5px dashed red;
padding: 9px
}
em {
outline: 5px dashed grey;
padding: 6px
}
u {
outline: 5px solid black;
padding: 3px;
}
u::before {
content: 'THIS TEXT SHOULD BE UNDERLINED'
}
const frag = document.createDocumentFragment();
const useless = ["ul", "li", "strong", "em", "u"];
let previous;
for (let u = 0; u < useless.length; u++) {
const current = document.createElement(useless[u]);
if (u === 0) {
frag.appendChild(current);
} else {
previous.appendChild(current);
}
previous = current;
}
document.body.appendChild(frag);
ul {
outline: 5px dashed green;
padding: 15px;
}
li {
outline: 5px solid blue;
padding: 12px;
}
strong {
outline: 5px dashed red;
padding: 9px
}
em {
outline: 5px dashed grey;
padding: 6px
}
u {
outline: 5px solid black;
padding: 3px;
}
u::before {
content: 'THIS TEXT SHOULD BE UNDERLINED'
}
answered 2 days ago
zer00nezer00ne
25k32545
25k32545
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55311908%2fcreating-nested-elements-dynamically%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
i3t,W8rdB
1
You want to know about
Node.appendChild
.– moonwave99
2 days ago