Python scanner for the first free port in a range
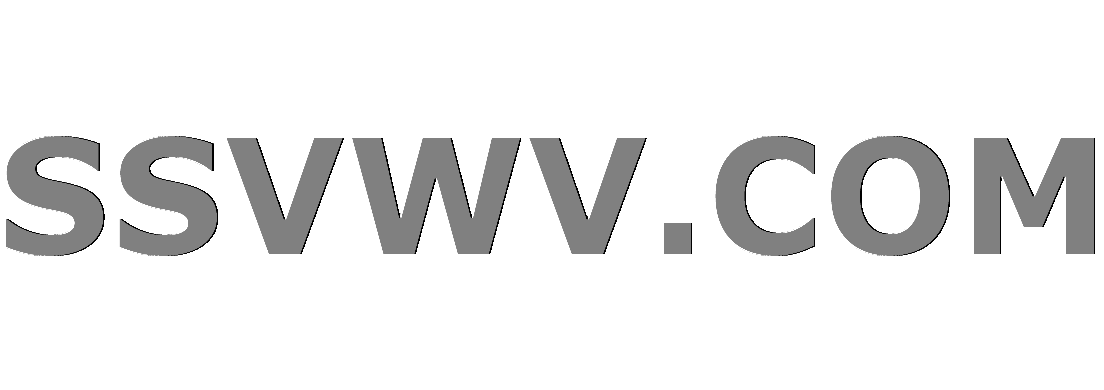
Multi tool use
$begingroup$
I have a distributed application (YARN), which runs a WebApp.
This application use a default port to start (8008), before I start I need to check if port is in use.
A container may run in the same virtual machine, hence port may be in use.
(Max I have 4 containers in WebApp).
I created the following code which seem to work, but want to see if there are some clean ups/improvements suggested.
def port_in_use(port):
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
result = sock.connect_ex(('127.0.0.1', port))
if result == 0:
return True
else:
return False
def start_dashboard():
base_port = os.getenv('DASHBOARD_PORT_ENV_VAR', 8008)
scan_ports = True
attempts = 0
max_attempts = 10
while(scan_ports and attempts <= max_attempts):
if port_in_use(base_port):
base_port += 1
attempts += 1
else:
scan_ports = False
if attempts == max_attempts:
raise IOError('Port in use')
dashboard.configure(port=base_port)
dashboard.launch()
python python-3.x networking socket
$endgroup$
add a comment |
$begingroup$
I have a distributed application (YARN), which runs a WebApp.
This application use a default port to start (8008), before I start I need to check if port is in use.
A container may run in the same virtual machine, hence port may be in use.
(Max I have 4 containers in WebApp).
I created the following code which seem to work, but want to see if there are some clean ups/improvements suggested.
def port_in_use(port):
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
result = sock.connect_ex(('127.0.0.1', port))
if result == 0:
return True
else:
return False
def start_dashboard():
base_port = os.getenv('DASHBOARD_PORT_ENV_VAR', 8008)
scan_ports = True
attempts = 0
max_attempts = 10
while(scan_ports and attempts <= max_attempts):
if port_in_use(base_port):
base_port += 1
attempts += 1
else:
scan_ports = False
if attempts == max_attempts:
raise IOError('Port in use')
dashboard.configure(port=base_port)
dashboard.launch()
python python-3.x networking socket
$endgroup$
add a comment |
$begingroup$
I have a distributed application (YARN), which runs a WebApp.
This application use a default port to start (8008), before I start I need to check if port is in use.
A container may run in the same virtual machine, hence port may be in use.
(Max I have 4 containers in WebApp).
I created the following code which seem to work, but want to see if there are some clean ups/improvements suggested.
def port_in_use(port):
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
result = sock.connect_ex(('127.0.0.1', port))
if result == 0:
return True
else:
return False
def start_dashboard():
base_port = os.getenv('DASHBOARD_PORT_ENV_VAR', 8008)
scan_ports = True
attempts = 0
max_attempts = 10
while(scan_ports and attempts <= max_attempts):
if port_in_use(base_port):
base_port += 1
attempts += 1
else:
scan_ports = False
if attempts == max_attempts:
raise IOError('Port in use')
dashboard.configure(port=base_port)
dashboard.launch()
python python-3.x networking socket
$endgroup$
I have a distributed application (YARN), which runs a WebApp.
This application use a default port to start (8008), before I start I need to check if port is in use.
A container may run in the same virtual machine, hence port may be in use.
(Max I have 4 containers in WebApp).
I created the following code which seem to work, but want to see if there are some clean ups/improvements suggested.
def port_in_use(port):
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
result = sock.connect_ex(('127.0.0.1', port))
if result == 0:
return True
else:
return False
def start_dashboard():
base_port = os.getenv('DASHBOARD_PORT_ENV_VAR', 8008)
scan_ports = True
attempts = 0
max_attempts = 10
while(scan_ports and attempts <= max_attempts):
if port_in_use(base_port):
base_port += 1
attempts += 1
else:
scan_ports = False
if attempts == max_attempts:
raise IOError('Port in use')
dashboard.configure(port=base_port)
dashboard.launch()
python python-3.x networking socket
python python-3.x networking socket
edited 2 days ago


200_success
130k17155419
130k17155419
asked 2 days ago
spicyramenspicyramen
307312
307312
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
$begingroup$
Your code has some incorrect assumptions.
an application may listen on a specific address/port combination;
127.0.0.1:port
can be available while*:port
is not.an application may bind a port without listening. Connects will fail, but so will your own bind.
a firewall or other mechanism can interfere with connections, generating false positives in your scan.
The reliable approach is to bind the port, just as your dashboard will, and then release it.
result = sock.bind(('', port))
sock.close()
You'll need to catch the exception and this is a good opportunity to move the whole thing into a function. That will make the start_dashboard
logic cleaner and get rid of boolean loop-terminator scan_ports
. Just exit the loop by return
ing the answer.
def next_free_port( port=1024, max_port=65535 ):
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
while port <= max_port:
try:
sock.bind(('', port))
sock.close()
return port
except OSError:
port += 1
raise IOError('no free ports')
def start_dashboard():
# pass optional second parameter "max_port" here, else scan until a free one is found
port = next_free_port( os.getenv('DASHBOARD_PORT_ENV_VAR', 8008) )
dashboard.configure(port=port)
dashboard.launch()
You can use netcat to make ports in-use for testing: nc -l -p 9999
will listen on port 9999; press control-C to end it.
$endgroup$
$begingroup$
Thank you for the answer, check is local, so no firewall in place.
$endgroup$
– spicyramen
2 days ago
$begingroup$
I mean a host firewall, like iptables on Linux.
$endgroup$
– Oh My Goodness
2 days ago
$begingroup$
Sounds good, any recommendation for Python style? Thanks
$endgroup$
– spicyramen
yesterday
1
$begingroup$
the code can be a little shorter and clearer; see edits for a fleshed-out example.
$endgroup$
– Oh My Goodness
yesterday
1
$begingroup$
Thank you! Great sample
$endgroup$
– spicyramen
yesterday
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f216037%2fpython-scanner-for-the-first-free-port-in-a-range%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
Your code has some incorrect assumptions.
an application may listen on a specific address/port combination;
127.0.0.1:port
can be available while*:port
is not.an application may bind a port without listening. Connects will fail, but so will your own bind.
a firewall or other mechanism can interfere with connections, generating false positives in your scan.
The reliable approach is to bind the port, just as your dashboard will, and then release it.
result = sock.bind(('', port))
sock.close()
You'll need to catch the exception and this is a good opportunity to move the whole thing into a function. That will make the start_dashboard
logic cleaner and get rid of boolean loop-terminator scan_ports
. Just exit the loop by return
ing the answer.
def next_free_port( port=1024, max_port=65535 ):
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
while port <= max_port:
try:
sock.bind(('', port))
sock.close()
return port
except OSError:
port += 1
raise IOError('no free ports')
def start_dashboard():
# pass optional second parameter "max_port" here, else scan until a free one is found
port = next_free_port( os.getenv('DASHBOARD_PORT_ENV_VAR', 8008) )
dashboard.configure(port=port)
dashboard.launch()
You can use netcat to make ports in-use for testing: nc -l -p 9999
will listen on port 9999; press control-C to end it.
$endgroup$
$begingroup$
Thank you for the answer, check is local, so no firewall in place.
$endgroup$
– spicyramen
2 days ago
$begingroup$
I mean a host firewall, like iptables on Linux.
$endgroup$
– Oh My Goodness
2 days ago
$begingroup$
Sounds good, any recommendation for Python style? Thanks
$endgroup$
– spicyramen
yesterday
1
$begingroup$
the code can be a little shorter and clearer; see edits for a fleshed-out example.
$endgroup$
– Oh My Goodness
yesterday
1
$begingroup$
Thank you! Great sample
$endgroup$
– spicyramen
yesterday
|
show 1 more comment
$begingroup$
Your code has some incorrect assumptions.
an application may listen on a specific address/port combination;
127.0.0.1:port
can be available while*:port
is not.an application may bind a port without listening. Connects will fail, but so will your own bind.
a firewall or other mechanism can interfere with connections, generating false positives in your scan.
The reliable approach is to bind the port, just as your dashboard will, and then release it.
result = sock.bind(('', port))
sock.close()
You'll need to catch the exception and this is a good opportunity to move the whole thing into a function. That will make the start_dashboard
logic cleaner and get rid of boolean loop-terminator scan_ports
. Just exit the loop by return
ing the answer.
def next_free_port( port=1024, max_port=65535 ):
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
while port <= max_port:
try:
sock.bind(('', port))
sock.close()
return port
except OSError:
port += 1
raise IOError('no free ports')
def start_dashboard():
# pass optional second parameter "max_port" here, else scan until a free one is found
port = next_free_port( os.getenv('DASHBOARD_PORT_ENV_VAR', 8008) )
dashboard.configure(port=port)
dashboard.launch()
You can use netcat to make ports in-use for testing: nc -l -p 9999
will listen on port 9999; press control-C to end it.
$endgroup$
$begingroup$
Thank you for the answer, check is local, so no firewall in place.
$endgroup$
– spicyramen
2 days ago
$begingroup$
I mean a host firewall, like iptables on Linux.
$endgroup$
– Oh My Goodness
2 days ago
$begingroup$
Sounds good, any recommendation for Python style? Thanks
$endgroup$
– spicyramen
yesterday
1
$begingroup$
the code can be a little shorter and clearer; see edits for a fleshed-out example.
$endgroup$
– Oh My Goodness
yesterday
1
$begingroup$
Thank you! Great sample
$endgroup$
– spicyramen
yesterday
|
show 1 more comment
$begingroup$
Your code has some incorrect assumptions.
an application may listen on a specific address/port combination;
127.0.0.1:port
can be available while*:port
is not.an application may bind a port without listening. Connects will fail, but so will your own bind.
a firewall or other mechanism can interfere with connections, generating false positives in your scan.
The reliable approach is to bind the port, just as your dashboard will, and then release it.
result = sock.bind(('', port))
sock.close()
You'll need to catch the exception and this is a good opportunity to move the whole thing into a function. That will make the start_dashboard
logic cleaner and get rid of boolean loop-terminator scan_ports
. Just exit the loop by return
ing the answer.
def next_free_port( port=1024, max_port=65535 ):
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
while port <= max_port:
try:
sock.bind(('', port))
sock.close()
return port
except OSError:
port += 1
raise IOError('no free ports')
def start_dashboard():
# pass optional second parameter "max_port" here, else scan until a free one is found
port = next_free_port( os.getenv('DASHBOARD_PORT_ENV_VAR', 8008) )
dashboard.configure(port=port)
dashboard.launch()
You can use netcat to make ports in-use for testing: nc -l -p 9999
will listen on port 9999; press control-C to end it.
$endgroup$
Your code has some incorrect assumptions.
an application may listen on a specific address/port combination;
127.0.0.1:port
can be available while*:port
is not.an application may bind a port without listening. Connects will fail, but so will your own bind.
a firewall or other mechanism can interfere with connections, generating false positives in your scan.
The reliable approach is to bind the port, just as your dashboard will, and then release it.
result = sock.bind(('', port))
sock.close()
You'll need to catch the exception and this is a good opportunity to move the whole thing into a function. That will make the start_dashboard
logic cleaner and get rid of boolean loop-terminator scan_ports
. Just exit the loop by return
ing the answer.
def next_free_port( port=1024, max_port=65535 ):
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
while port <= max_port:
try:
sock.bind(('', port))
sock.close()
return port
except OSError:
port += 1
raise IOError('no free ports')
def start_dashboard():
# pass optional second parameter "max_port" here, else scan until a free one is found
port = next_free_port( os.getenv('DASHBOARD_PORT_ENV_VAR', 8008) )
dashboard.configure(port=port)
dashboard.launch()
You can use netcat to make ports in-use for testing: nc -l -p 9999
will listen on port 9999; press control-C to end it.
edited yesterday
answered 2 days ago


Oh My GoodnessOh My Goodness
1,809314
1,809314
$begingroup$
Thank you for the answer, check is local, so no firewall in place.
$endgroup$
– spicyramen
2 days ago
$begingroup$
I mean a host firewall, like iptables on Linux.
$endgroup$
– Oh My Goodness
2 days ago
$begingroup$
Sounds good, any recommendation for Python style? Thanks
$endgroup$
– spicyramen
yesterday
1
$begingroup$
the code can be a little shorter and clearer; see edits for a fleshed-out example.
$endgroup$
– Oh My Goodness
yesterday
1
$begingroup$
Thank you! Great sample
$endgroup$
– spicyramen
yesterday
|
show 1 more comment
$begingroup$
Thank you for the answer, check is local, so no firewall in place.
$endgroup$
– spicyramen
2 days ago
$begingroup$
I mean a host firewall, like iptables on Linux.
$endgroup$
– Oh My Goodness
2 days ago
$begingroup$
Sounds good, any recommendation for Python style? Thanks
$endgroup$
– spicyramen
yesterday
1
$begingroup$
the code can be a little shorter and clearer; see edits for a fleshed-out example.
$endgroup$
– Oh My Goodness
yesterday
1
$begingroup$
Thank you! Great sample
$endgroup$
– spicyramen
yesterday
$begingroup$
Thank you for the answer, check is local, so no firewall in place.
$endgroup$
– spicyramen
2 days ago
$begingroup$
Thank you for the answer, check is local, so no firewall in place.
$endgroup$
– spicyramen
2 days ago
$begingroup$
I mean a host firewall, like iptables on Linux.
$endgroup$
– Oh My Goodness
2 days ago
$begingroup$
I mean a host firewall, like iptables on Linux.
$endgroup$
– Oh My Goodness
2 days ago
$begingroup$
Sounds good, any recommendation for Python style? Thanks
$endgroup$
– spicyramen
yesterday
$begingroup$
Sounds good, any recommendation for Python style? Thanks
$endgroup$
– spicyramen
yesterday
1
1
$begingroup$
the code can be a little shorter and clearer; see edits for a fleshed-out example.
$endgroup$
– Oh My Goodness
yesterday
$begingroup$
the code can be a little shorter and clearer; see edits for a fleshed-out example.
$endgroup$
– Oh My Goodness
yesterday
1
1
$begingroup$
Thank you! Great sample
$endgroup$
– spicyramen
yesterday
$begingroup$
Thank you! Great sample
$endgroup$
– spicyramen
yesterday
|
show 1 more comment
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f216037%2fpython-scanner-for-the-first-free-port-in-a-range%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
X7PRit3P N4 89YIwO,t7rSpIWZtw6,wIF8 ZmyftoVHYq,Si4 T8YZDVmFClvorqGBZ,Y wO0,uY2hgdzdHPE7tuctUExwTT 4oNK,g0 M3O